Table of Contents
In today’s technology-driven world, the Internet of Things (IoT) has gained immense popularity. IoT devices, such as the Raspberry Pi, have become the cornerstone of various projects, from home automation to industrial applications. To make the most out of your Raspberry Pi, you might want to connect it to cloud services. This allows you to store, process, and analyze data remotely, opening up a world of possibilities. In this comprehensive guide, we’ll explore how to connect your Raspberry Pi to various cloud services using Python.
Why Connect Your Raspberry Pi to the Cloud?

Before we dive into the technical details, let’s discuss why you might want to connect your Raspberry Pi to the cloud. There are several compelling reasons:
1. Remote Access
By connecting your Raspberry Pi to the cloud, you can access and control it from anywhere with an internet connection. This is especially useful if your Raspberry Pi is in a different location from where you are.
2. Data Storage
Cloud services offer abundant storage space, allowing you to store sensor data, logs, or any other information your Raspberry Pi generates. This data can be accessed and analyzed at any time.
3. Scalability
Cloud platforms provide scalable resources, so you can easily adapt to increased data or computational requirements as your project grows.
4. Security
Cloud providers often have robust security measures in place, protecting your data from physical damage or unauthorized access.
5. Collaboration
If you’re working on a project with others, cloud services facilitate collaboration by allowing multiple users to access and work with the same data simultaneously.
Now that we understand the advantages, let’s move on to the technical aspects of connecting your Raspberry Pi to the cloud.
Choosing the Right Cloud Service
The first step in connecting your Raspberry Pi to the cloud is selecting the appropriate cloud service provider. There are several popular options to consider:
1. Amazon Web Services (AWS)

AWS offers a wide range of cloud services, including AWS IoT Core, which is designed for IoT applications. It provides MQTT-based communication and supports Python for device interactions.
2. Google Cloud Platform (GCP)
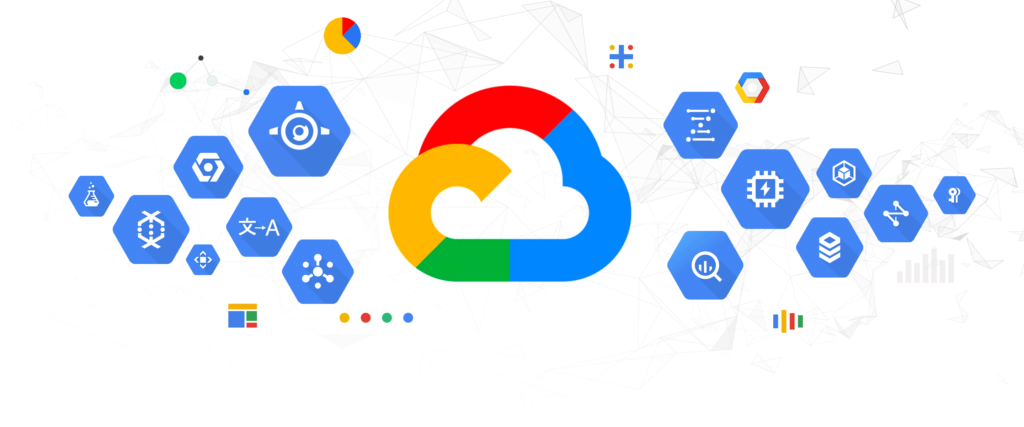
GCP provides IoT Core for device management and Cloud Pub/Sub for data ingestion. Python is well-supported for both.
3. Microsoft Azure
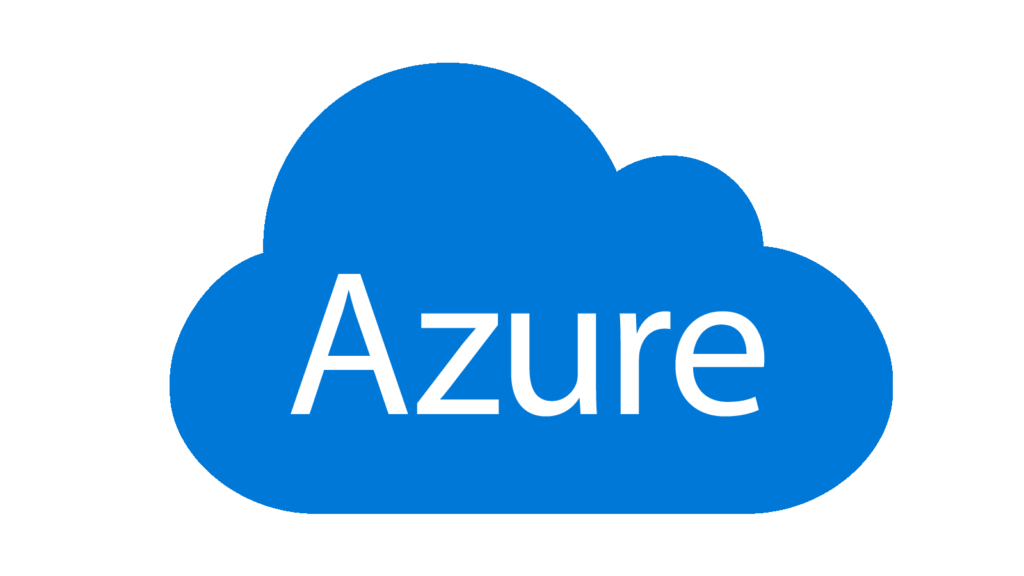
Azure IoT Hub is Microsoft’s solution for IoT device management and connectivity. You can use Azure Functions and Python for processing data.
4. IBM Cloud

IBM offers IoT Platform and Watson IoT for device management and analytics. Python can be used for device integration and data processing.
5. Other Cloud Services
Apart from the major players, there are many other cloud providers and platforms to explore, depending on your specific project requirements.
The choice of cloud service provider will depend on factors such as your familiarity with the platform, pricing, and the specific features you need for your project. Once you’ve chosen a cloud provider, you can proceed with connecting your Raspberry Pi.
Setting Up Your Raspberry Pi
Before you can connect your Raspberry Pi to the cloud, you’ll need to ensure that it’s set up and ready to go. Here are the basic steps:
1. Get a Raspberry Pi
If you don’t already have one, obtain a Raspberry Pi board (such as Raspberry Pi 4) and necessary accessories, including a power supply, microSD card, and a compatible operating system (Raspberry Pi OS is a popular choice).
2. Connect to the Internet
Ensure that your Raspberry Pi is connected to the internet, either via Ethernet or Wi-Fi. You’ll need internet access to communicate with the cloud.
3. Install Python
Raspberry Pi typically comes with Python pre-installed, but you may need to update it to the latest version or install additional packages depending on your project requirements.
4. Set Up SSH
If you plan to access your Raspberry Pi remotely, set up SSH (Secure Shell) for secure command-line access.
With your Raspberry Pi prepared, we’re now ready to start connecting it to the cloud.
Part 2: Connecting Your Raspberry Pi to AWS IoT Core with Python
In Part 2 of our guide, we’ll delve into the specifics of connecting your Raspberry Pi to AWS IoT Core, one of the popular cloud services for IoT applications. We’ll also explore how to use Python to interact with AWS IoT Core.
What is AWS IoT Core?
AWS IoT Core is a managed cloud service provided by Amazon Web Services (AWS) that is specifically designed for connecting and managing IoT devices securely. It allows you to communicate with and control IoT devices over MQTT (Message Queuing Telemetry Transport) and HTTP protocols.
Here are some key features of AWS IoT Core:
- Device Management: AWS IoT Core provides tools for registering, managing, and organizing your IoT devices.
- Message Broker: It includes a message broker that enables devices to publish messages (data) and subscribe to topics to receive messages.
- Security: Security is a top priority. AWS IoT Core offers authentication and authorization mechanisms to ensure that only authorized devices can interact with your IoT resources.
- Rules Engine: You can define rules to process and route data from your devices to various AWS services such as Amazon S3, DynamoDB, or Lambda functions.
Connecting Your Raspberry Pi to AWS IoT Core
To connect your Raspberry Pi to AWS IoT Core, follow these steps:
Step 1: Create an AWS Account
If you don’t have an AWS account, sign up for one at AWS Console. You may need to provide billing information, but AWS offers a free tier with limited usage, which is suitable for getting started.
Step 2: Set Up AWS IoT Core
- Log in to your AWS account and navigate to the AWS IoT Core console.
- Create a new “Thing” to represent your Raspberry Pi. This will generate a unique Thing ARN (Amazon Resource Name).
- Generate and download security certificates and keys for your Raspberry Pi. You’ll need these to establish a secure connection. Make sure to keep them safe and don’t share them publicly.
Step 3: Configure Your Raspberry Pi
- Install the AWS IoT SDK for Python (Boto3) on your Raspberry Pi using
pip
: pip install boto3 - Use the AWS security certificates and keys you downloaded earlier to configure your Raspberry Pi’s connection to AWS IoT Core. import boto3 import ssl from AWSIoTPythonSDK.MQTTLib import AWSIoTMQTTClient AWS IoT Core endpoint host = “your-iot-endpoint.amazonaws.com” root_ca = “root-CA.pem” private_key = “your-private-key.pem.key” certificate = “your-certificate.pem.crt” Initialize the AWS IoT MQTT Client client = AWSIoTMQTTClient(“RaspberryPi”) client.configureEndpoint(host, 8883) client.configureCredentials(root_ca, private_key, certificate) Connect to AWS IoT Core client.connect() Subscribe to a topic client.subscribe(“myTopic”, 1, callback) Publish a message client.publish(“myTopic”, “Hello, AWS IoT Core!”, 1) Define a callback function for incoming messages def callback(client, userdata, message):
print(f"Received message '{message.payload}' on topic '{message.topic}'")
Keep the script running while True:pass
Step 4: Test the Connection
Run the Python script on your Raspberry Pi. It should connect to AWS IoT Core, subscribe to a topic, and be able to send and receive messages.
Congratulations! Your Raspberry Pi is now connected to AWS IoT Core, and you can start sending and receiving data securely.
Part 3: Interacting with AWS IoT Core Using Python on Your Raspberry Pi
In Part 3 of our guide, we’ll explore how to interact with AWS IoT Core using Python. We’ll cover how to send and receive data from your Raspberry Pi to the cloud and explore some practical use cases.
Sending Data to AWS IoT Core
To send data from your Raspberry Pi to AWS IoT Core, you can use the AWS IoT SDK for Python (Boto3). We’ll expand on the Python script from Part 2 to publish data to AWS IoT Core.
Here’s how you can modify the script to send data:
import boto3
import ssl
from AWSIoTPythonSDK.MQTTLib import AWSIoTMQTTClient
# AWS IoT Core endpoint
host = "your-iot-endpoint.amazonaws.com"
root_ca = "root-CA.pem"
private_key = "your-private-key.pem.key"
certificate = "your-certificate.pem.crt"
# Initialize the AWS IoT MQTT Client
client = AWSIoTMQTTClient("RaspberryPi")
client.configureEndpoint(host, 8883)
client.configureCredentials(root_ca, private_key, certificate)
# Connect to AWS IoT Core
client.connect()
# Define a callback function for incoming messages
def callback(client, userdata, message):
print(f"Received message '{message.payload}' on topic '{message.topic}'")
# Define the topic to publish to
publish_topic = "myTopic"
# Send data to AWS IoT Core
data_to_send = "Sensor reading: 25�C"
client.publish(publish_topic, data_to_send, 1)
# Keep the script running
while True:
pass
In this script, we’ve added the ability to publish data to the “myTopic” topic. You can replace “Sensor reading: 25 degree C” with the actual data you want to send from your Raspberry Pi. This data could be sensor readings, status updates, or any other information relevant to your IoT project.
Receiving Data from AWS IoT Core
To receive data from AWS IoT Core on your Raspberry Pi, you can subscribe to a topic and define a callback function to process incoming messages, as demonstrated in the previous parts. Here’s a quick reminder of the relevant code:
# Subscribe to a topic
client.subscribe("myTopic", 1, callback)
# Define a callback function for incoming messages
def callback(client, userdata, message):
print(f"Received message '{message.payload}' on topic '{message.topic}'")
Whenever a message is published to the “myTopic” topic, the callback
function will be triggered, allowing you to process the incoming data. You can customize the callback function to suit your project’s needs.
Use Cases for AWS IoT Core
AWS IoT Core opens up numerous possibilities for IoT projects on your Raspberry Pi. Here are a few use cases:
1. Temperature Monitoring
Use a temperature sensor connected to your Raspberry Pi to monitor and send temperature readings to AWS IoT Core. You can then create alerts or trigger actions based on temperature thresholds.
2. Home Automation
Control and monitor smart devices in your home using your Raspberry Pi. For example, turn lights on and off remotely or receive alerts when motion is detected.
3. Industrial Monitoring
Implement predictive maintenance in industrial settings by collecting data from machines and using AWS IoT Core to analyze it for signs of potential issues.
4. Environmental Monitoring
Deploy your Raspberry Pi in remote locations to monitor environmental parameters like air quality, humidity, and soil conditions.
5. Security Systems
Create a home security system using cameras and sensors connected to your Raspberry Pi. Stream video footage to the cloud and receive alerts for suspicious activity.
AWS IoT Core’s integration with other AWS services allows you to build complex IoT applications with ease. In Part 4 of this guide, we’ll explore how to integrate AWS IoT Core with other AWS services to unlock even more capabilities for your Raspberry Pi IoT project.
Stay tuned for the final part of our guide to connecting your Raspberry Pi to various cloud services using Python!
Part 4: Integrating AWS IoT Core with Other AWS Services
In Part 4 of our guide, we’ll explore how to integrate AWS IoT Core with other AWS services. This integration allows you to leverage the power of the cloud for data processing, storage, and more.
Why Integrate with Other AWS Services?
Integrating AWS IoT Core with other AWS services opens up a wide range of possibilities for your Raspberry Pi IoT project:
- Data Storage: Store the data sent from your Raspberry Pi in Amazon S3 buckets for long-term storage and easy access.
- Data Analysis: Use Amazon Kinesis for real-time data streaming and analytics or leverage AWS Lambda functions to process incoming data.
- Database Management: Store data in Amazon DynamoDB for fast and scalable database storage or use Amazon RDS for relational database needs.
- Visualization: Create interactive dashboards using Amazon QuickSight or third-party tools like Grafana to visualize data collected from your Raspberry Pi.
- Alerting: Set up alarms and notifications using Amazon SNS (Simple Notification Service) to receive alerts based on specific conditions or thresholds.
- Machine Learning: Apply machine learning models to your data using Amazon SageMaker for predictive analytics or custom AI solutions.
Integrating AWS IoT Core with Other AWS Services
To integrate AWS IoT Core with other AWS services, follow these steps:
Step 1: Set Up the Integration
- AWS IoT Rules: In the AWS IoT Core console, create an IoT rule. This rule defines the conditions under which incoming messages should be processed and routed to other AWS services. For example, you can create a rule to store all temperature readings above a certain threshold in an S3 bucket.
- AWS IAM Roles: Create IAM roles that allow AWS IoT Core to access other AWS services. These roles define the permissions needed for data processing, storage, and more.
Step 2: Define Actions
For each IoT rule you create, define one or more actions. Actions determine what happens when a message matches the conditions specified in the rule. Here are some common actions:
- Store in Amazon S3: Save the message payload (data) in an Amazon S3 bucket for long-term storage.
- Invoke AWS Lambda: Trigger a Lambda function to process and analyze the incoming data. You can use Python in your Lambda functions.
- Write to Amazon DynamoDB: Store the data in a DynamoDB table for structured storage and retrieval.
- Send Notifications: Use Amazon SNS to send notifications or alerts based on specific conditions in the data.
Step 3: Test the Integration
Once you’ve configured the IoT rule and actions, test the integration by sending data from your Raspberry Pi to AWS IoT Core. Verify that the data is being processed and routed to the specified AWS services.
Step 4: Monitor and Scale
Monitor the integration to
ensure that data is flowing smoothly and that your AWS services are functioning as expected. You can set up alarms and logging to track the performance of your IoT project.
Practical Examples
Here are a few practical examples of what you can achieve with AWS IoT Core integration:
1. Historical Data Storage
Store sensor data from your Raspberry Pi in an S3 bucket for historical analysis. You can later use Amazon Athena to query and analyze this data.
2. Real-time Alerts
Set up AWS Lambda functions to analyze sensor data in real-time and trigger notifications if values exceed thresholds.
3. Dashboard Creation
Use Amazon QuickSight to create interactive dashboards displaying real-time data from your Raspberry Pi.
4. Predictive Maintenance
Implement machine learning models with Amazon SageMaker to predict when equipment, such as motors or pumps, might fail based on sensor data.
Remember to configure your IoT project according to your specific requirements and security best practices, including proper IAM policies and access control.
Conclusion
In conclusion, connecting your Raspberry Pi to various cloud services using Python opens up a world of possibilities for IoT projects. Whether you’re monitoring environmental conditions, controlling smart devices, or conducting industrial analysis, the cloud empowers your Raspberry Pi with scalability, data processing capabilities, and endless oppo
rtunities for innovation. By integrating AWS IoT Core with other AWS services, you can take your Raspberry Pi IoT project to new heights and create solutions that are limited only by your imagination.
Leave a Reply